Getting Started - Clients
ServiceStack Clients
As ServiceStack is just a pure HTTP web service it can be accessed with any HTTP-capable client. ServiceStack libraries provide out of the box client libraries for all of most popular platforms and exposes a consistent and intuitive client API that takes advantage of the message centric design of ServiceStack services.
This supports developers to wrap their own web service APIs in their custom client SDK with minimal effort since underlying ServiceStack libraries can be used as a base.
This is an especially good idea if you want to support static languages (i.e. C# and Java) where having typed client libraries saves end-users from reverse engineering the types and API calls. It also saves them having to look up documentation since a lot of it can be inferred from the type info.
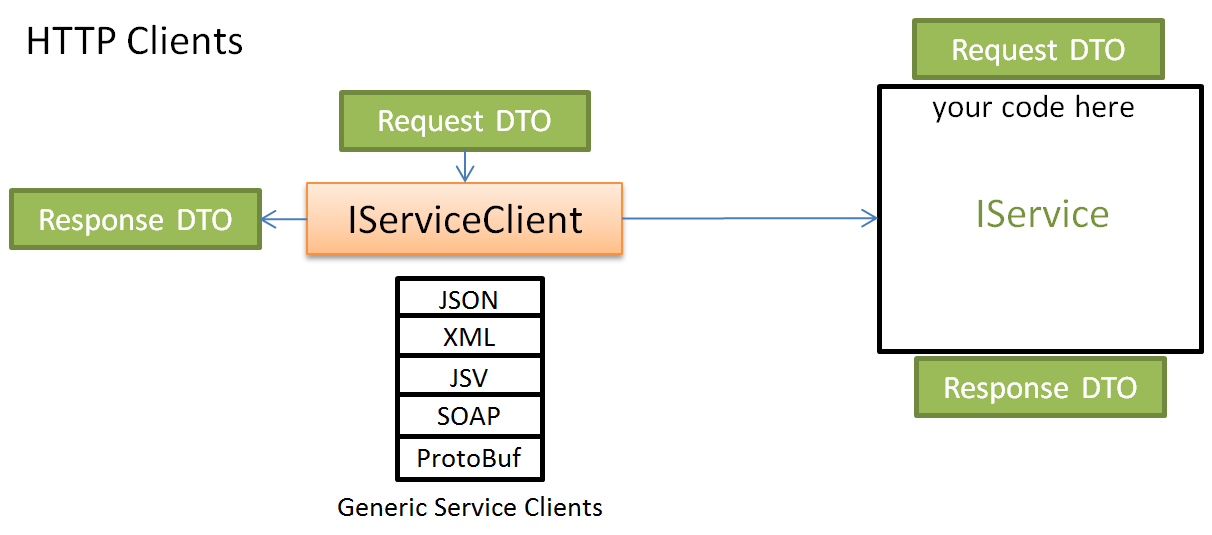
Service Clients
Using DTOs to define your web service interface makes it possible to provide strong-typed generic service clients without any code-gen or extra build-steps, leading to a productive end-to-end type-safe communication gateway from client to server.
This experience is consistent across ServiceStack client libraries and DTOs can be easily shared through tooling like Add ServiceStack Reference. These clients are optimized for consuming ServiceStack Services including built-in Error handling, Predefined Routes, Auto Batched Requests, etc.
var baseUrl = "https://web.web-templates.io";
var client = new JsonServiceClient(baseUrl);
var response = client.Get(new Hello { Name = "World!" });
Dart
import 'package:servicestack/client.dart';
import 'dtos.dart';
var baseUrl = "https://web.web-templates.io";
var client = new JsonServiceClient(baseUrl);
var response = await client.get(new Hello(name:"World!"));
Java
String baseUrl = "https://web.web-templates.io";
JsonServiceClient client = new JsonServiceClient(baseUrl);
HelloResponse = client.get(new Hello().setName("World!"));
Swift
var baseUrl = "https://web.web-templates.io";
var client = JsonServiceClient(baseUrl:baseUrl)
var request = Hello()
request.name = "World!"
let response = client.get(request);
TypeScript
import { JsonServiceClient } from 'servicestack-client'
import { Hello } from './web.dtos'
let baseUrl = "https://web.web-templates.io"
let client = new JsonServiceClient(baseUrl)
let request = new Hello({ name: 'World!' })
let response = await client.get(request) //res:HelloResponse
Web Client
TypeScript provides a great developer experience for web developers, the additional type information and safety also makes ServiceStack's typed end to end services a pleasure to use.
The NPM library @servicestack/client
combined with your service DTOs which are generated by ServiceStack takes the guess work out of what data structures will be returning from each service.
Here, in a VueJS project, we can see the server DTOs in C# as well as what the TypeScript DTOs that the server generates from them. The Service Client can infer the response type from the request type thanks to the use of IReturn<T>.
This provides web development with autocomplete of the available requests as well as typed objects in the response so you know exactly what data is available and it is typed without any additional effort.
[Route("/hello")]
[Route("/hello/{Name}")]
public class Hello : IReturn<HelloResponse>
{
public string Name { get; set; }
}
public class HelloResponse
{
public string Result { get; set; }
}
public class MyServices : Service
{
public object Any(Hello request) =>
new HelloResponse {
Result = $"Hello, {request.Name}!"
};
}
HelloApi.mjs
import { ref } from "vue"
import { useClient } from "@servicestack/vue"
import { Hello } from "/dtos.mjs"
export default {
template:/*html*/`<div class="flex flex-wrap justify-center">
<TextInput v-model="name" @keyup="update" />
<div class="ml-3 mt-2 text-lg">{{ result }}</div>
</div>`,
props:['value'],
setup(props) {
let name = ref(props.value)
let result = ref('')
let client = useClient()
async function update() {
let api = await client.api(new Hello({ name }))
if (api.succeeded) {
result.value = api.response.result
}
}
update()
return { name, update, result }
}
}
dtos.ts
export class HelloResponse
{
public result: string;
public constructor(init?: Partial<HelloResponse>) {
(Object as any).assign(this, init);
}
}
export class Hello implements IReturn<HelloResponse>
{
public name: string;
public constructor(init?: Partial<Hello>) {
(Object as any).assign(this, init);
}
public createResponse() { return new HelloResponse(); }
public getTypeName() { return 'Hello'; }
}
Native Clients
As well as supporting the use of cross platform frameworks like Xamarin, ServiceStack supports building native client apps through the array of service client libraries and tooling.
This means you'll have the most flexibility when it comes to building your client applications when integrating with a ServiceStack backend. You can use Swift
for iOS, Java
or Kotlin
for Android and get the most out of platform you are targeting.
The DTOs are live generated from a running ServiceStack server and can be viewed via the URL /types/{Name}
. Test this live using one of our hosted demo applications below.
public object Any(Hello request)
{
return new HelloResponse {
Result = $"Hello, {request.Name}!"
};
}
}
[Route("/hello")]
[Route("/hello/{Name}")]
public class Hello : IReturn<HelloResponse>
{
public string Name { get; set; }
}
public class HelloResponse
{
public string Result { get; set; }
}
dtos.kt
open class Hello : IReturn<HelloResponse>
{
var name:String? = null
companion object {
private val responseType =
HelloResponse::class.java
}
override fun getResponseType(): Any? =
Hello.responseType
}
open class HelloResponse
{
var result:String? = null
}
dtos.swift
public class Hello : IReturn, Codable
{
public typealias Return =
HelloResponse
public var name:String?
required public init(){}
}
public class HelloResponse : Codable
{
public var result:String?
required public init(){}
}
Cross platform tooling
The ability to generate DTOs from your ServiceStack server can be done with a simple, cross-platform CLI as well as with many populate IDEs via direct integration.
Adding integration only needs the base URL of the ServiceStack server, and a specified language. For example, if you were working on a TypeScript React application and wanted to integrate with a ServiceStack service, you could use the command.
x typescript https://web.web-template.io
This would generate the server DTOs in TypeScript, allowing you to easily communicate with the server using @servicestack/client
library. The same workflow works for all supported languages!
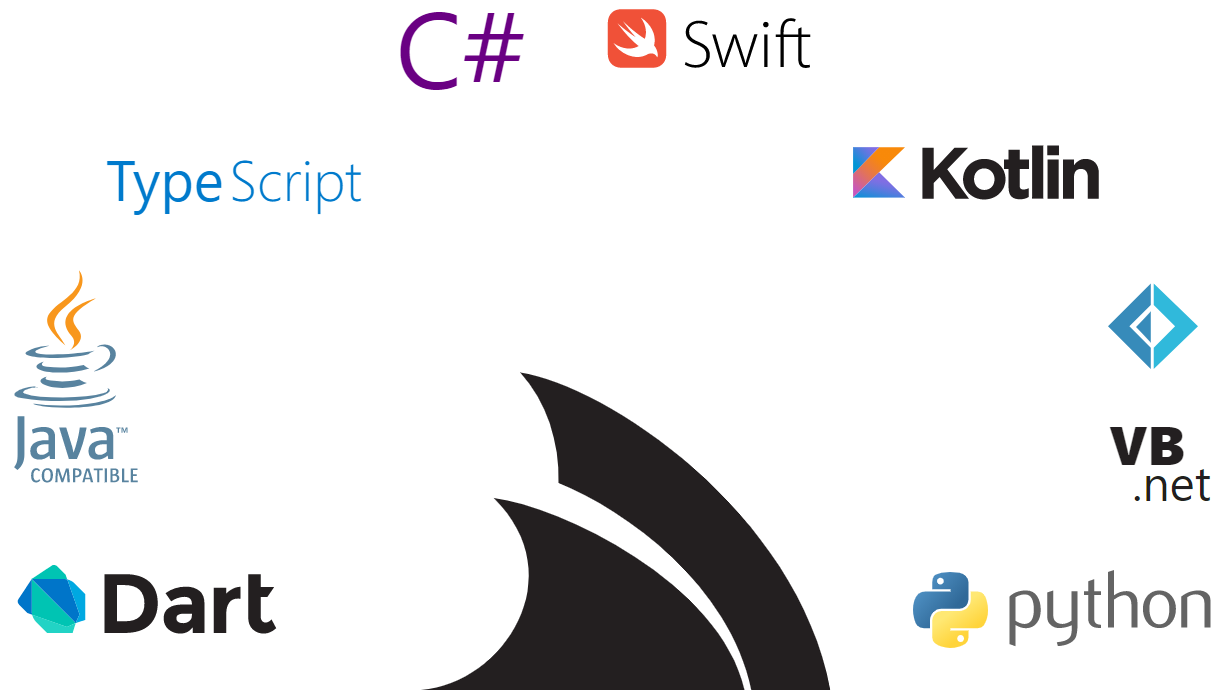
Instant Client Apps
The typed end to end clients also means we can generate complete working client application from a ServiceStack server. This enabled ServiceStack to offer a free web hosted tool to do just that and allow anyone to generate native client apps instantly with just a base URL.
Check out this video to see how this can be used to speed up client application development using Dart and Flutter!